Automating Workflows: Uploading to S3
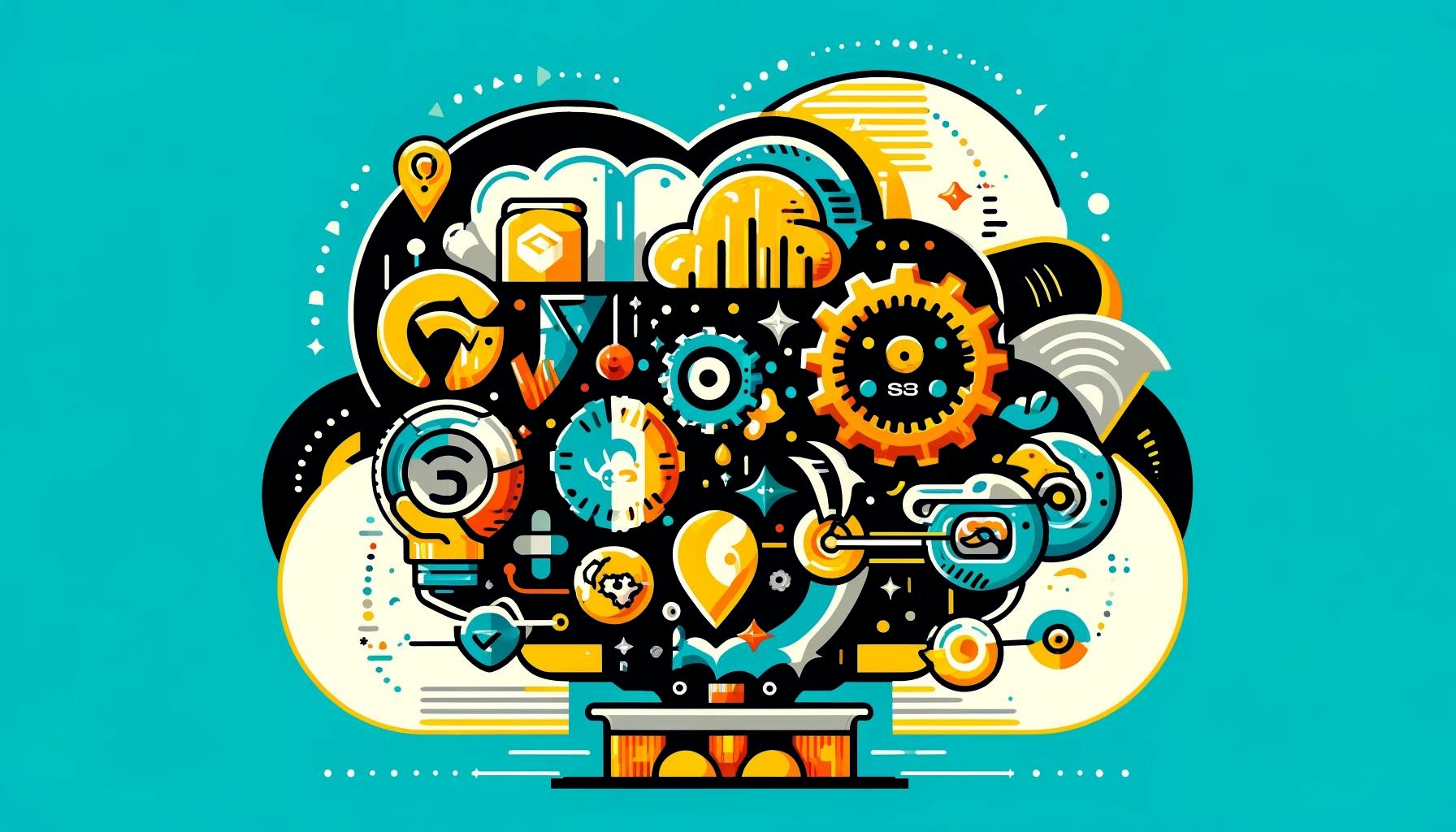
In this blog, we will explore three methods to automate the process of uploading your GitHub repository to an S3 bucket: GitHub Actions, AWS CodePipeline, and custom Git hooks. Each method is suited for different needs and levels of complexity.
1. GitHub Actions
GitHub Actions is a powerful tool to automate workflows directly within GitHub. You can set up a workflow to upload your repository to S3 after a push.
Steps:
- Create a
.github/workflows
directory in your repository. - Add a new workflow file, e.g.,
deploy.yml
, with the following content:
name: Deploy to S3
on:
push:
branches:
- main
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Configure AWS credentials
uses: aws-actions/configure-aws-credentials@v1
with:
aws-access-key-id: ${{ secrets.AWS_ACCESS_KEY_ID }}
aws-secret-access-key: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
aws-region: us-east-1
- name: Sync to S3
run: |
aws s3 sync . s3://your-s3-bucket-name --delete
- Add your AWS credentials (AWS_ACCESS_KEY_ID and AWS_SECRET_ACCESS_KEY) as secrets in your GitHub repository settings.
2. AWS CodePipeline
AWS CodePipeline is a fully managed continuous delivery service that helps automate your release pipelines for fast and reliable application and infrastructure updates.
Steps:
- Set up a CodePipeline with a source stage linked to your GitHub repository.
- Add a build stage using AWS CodeBuild to package your repository.
- Add a deploy stage to sync the repository to your S3 bucket using the
aws s3 sync
command.
3. Custom Git Hook
A Git hook can be set up to trigger a script after a push to the main branch, which can upload files to S3.
Steps:
- Create a
post-receive
hook in your Git repository on the server.
#!/bin/bash
# Navigate to the repository directory
cd /path/to/your/repo
# Sync the repository to S3
aws s3 sync . s3://your-s3-bucket-name --delete
- Make the script executable:
chmod +x /path/to/your/repo/.git/hooks/post-receive
- Ensure the script is run by the correct user with appropriate AWS credentials configured.
Summary
- GitHub Actions: Automate directly within GitHub with a simple YAML file. Requires GitHub credentials and setup.
- AWS CodePipeline: Use AWS's fully managed service for continuous delivery. Requires AWS setup and configuration.
- Custom Git Hook: Directly run scripts on your Git server to sync to S3. Requires access to the server and AWS CLI setup.
Each method has its own setup complexity and use case suitability, so choose the one that best fits your workflow.
- GitHub Actions: Ideal for those already using GitHub and looking for a straightforward way to automate workflows without leaving the GitHub environment. This method is relatively easy to set up but requires understanding GitHub's secret management for credentials.
- AWS CodePipeline: Best for those who need a fully managed, scalable solution integrated with other AWS services. It's more complex to set up initially due to the AWS configuration requirements but offers extensive capabilities for managing deployment pipelines.
- Custom Git Hook: Suitable for those who have access to their Git server and prefer direct control over the deployment process. This method is flexible and powerful but requires server access and proper configuration of the AWS CLI.